In this tutorial, we will show you how to use JEditorPane class to create a simple web browser that displays simple HTML documents.
JEditorPane is a kind of text area that can display various text formats. By default, JEditorPane supports HTML and RTF (Rich Text Format). However, you can build your own “editor kits” to handle specific content types. You can use setContentType() method to choose the document you want to display and setEditorKit() method to set custom editor for JEditorPane explicitly.
In practice, JEditorPane is typically used for displaying HTML only. JEditorPane also supports RTF but very limited. You can set content for JEditorPane in the following ways:
- Pass URL object or URL string into the constructor of JEditorPane.
- Use setPage() method to set content of JEditorPane at runtime.
- Pass content as a String to the setText() method.
- Use read() and supplying an HTMLDocument object with InputStream object.
To make JEditorPane read-only, you use method setEditable(false). When displaying HTML document, JEditorPane can detect HTML links and which link user clicks. To handle the click event on links, you need to handle HyperlinkListener event.
Example of using JEditorPane to create a simple web browser
In this example, we will create a very simple web browser to display any web page by using JEditorPane component. In this example, we are also using JButton and JTextField components.
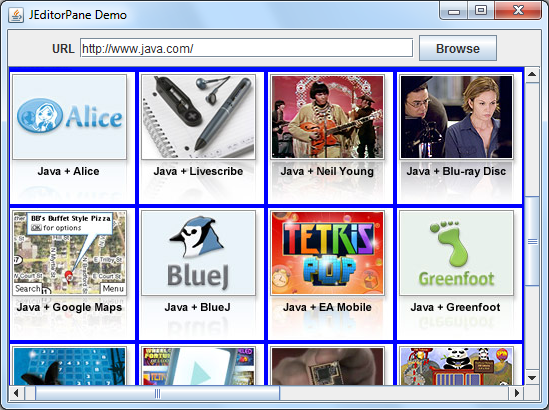
package jeditorpanedemo; import java.awt.*; import java.awt.event.*; import java.io.*; import javax.swing.*; import javax.swing.event.*; import javax.swing.text.html.*; public class Main { public static void main(String[] args) { final JFrame frame = new JFrame("JEditorPane Demo"); String initialURL = "http://www.java.com/"; final JEditorPane ed; JLabel lblURL = new JLabel("URL"); final JTextField txtURL = new JTextField(initialURL, 30); JButton btnBrowse = new JButton("Browse"); JPanel panel = new JPanel(); panel.setLayout(new FlowLayout()); panel.add(lblURL); panel.add(txtURL); panel.add(btnBrowse); try { ed = new JEditorPane(initialURL); ed.setEditable(false); ed.addHyperlinkListener(new HyperlinkListener() { public void hyperlinkUpdate(HyperlinkEvent e) { if (e.getEventType() == HyperlinkEvent.EventType.ACTIVATED) { JEditorPane pane = (JEditorPane) e.getSource(); if (e instanceof HTMLFrameHyperlinkEvent) { HTMLFrameHyperlinkEvent evt = (HTMLFrameHyperlinkEvent) e; HTMLDocument doc = (HTMLDocument) pane.getDocument(); doc.processHTMLFrameHyperlinkEvent(evt); } else { try { pane.setPage(e.getURL()); } catch (Throwable t) { t.printStackTrace(); } } } } }); btnBrowse.addActionListener( new ActionListener() { public void actionPerformed(ActionEvent e) { try { ed.setPage(txtURL.getText().trim()); } catch (IOException ex) { ex.printStackTrace(); } } }); JScrollPane sp = new JScrollPane(ed); frame.setLayout(new BorderLayout()); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.getContentPane().add(panel, BorderLayout.NORTH); frame.getContentPane().add(sp, BorderLayout.CENTER); frame.setSize(500, 350); frame.setVisible(true); } catch (IOException ex) { ex.printStackTrace(); } } }